Introduction
I wasn't having much luck finding a good simple example application that uses JPA, EJB3, and Maven2,
so I figured I'd create a simple skeleton application
that hopefully others will find useful. This blog entry http://famvdploeg.com/blog/?p=41
along with the Better Builds With Maven pdf (just google it) got me started. (I'm still new to Maven so I'm not sure I'm doing everything correct, so those with more experience please let me know
if there are modifications I should make.)
Setup and Running The Demo On JBoss
The final ear that is generated should run in most application servers. You might have to add a speicfic xxx-web.xml file for your particular
server in the web module and if your underlying ORM framework is not hibernate, you'll need to modify the persistence.xml in the
ejb module. This tutorial will describe the setup using JBoss5, but it shouldn't take much to have it working in Glassfish, OC4J, etc
NOTE ABOUT TESTING: mvn install will run the simple unit test. It also uses a log4j.properties file in test/resources and will output a log file ejb-jar-testing.log inside the lt-ejb module root. The test results will show up in lt-ear/target/surefire-reports. If you want to generate a nicer report, type mvn surefire-report:report from the command line. This will generate an html report called surefire-report.html under lt-ejb/target/site directory. (Running mvn site should generate that file as well, including a larger more complete report. See mvn docs for more info.)
- Assumes jdk5 or greater is installed and your JAVA_HOME environment variable set.
- Download and extract Maven2
- Set an environment variable MAVEN_HOME that points to your installation directory. Also add MAVEN_HOME/bin to your path.
- Download JBoss5 (at the time of this writing when you click on the Jboss5 download link you'll have an option of downloading a jdk6 version or a standard version. If you are using jdk6 you can use the jdk6 version, otherwise use the standard release. Code for this example was compiled with java5.)
- Set an environment variable JBOSS_HOME to your installation directory.
- Unzip the learntechnology-maven-jee source code (From now on this location will be refered to as the "project-dir")
- In our project-dir/external-resources directory there is a jboss-log4j.xml file. Backup your JBOSS_HOME/server/default/conf/jboss-log4j.xml file and replace it with this one. (Or you can just use the new appender and category that you see in the provided file to your existing jboss-log4j.xml file.)
-
Set up HSQLDB.
JBoss comes with its own version of HSQLDB, but I'll explain setting up hsqldb as an external db and we'll start it outside of JBoss as well. (Obviously you can use any other database you want, but you'll want to modify the learntechnology-ds.xml file accordingly and you'll need to take a look at the mydb.script to view the simple table created and the few inserts.)
- Download HSQLDB and extract to your directory of choice.
- Put the mysql.script (included in this project's external-resources directory) in the hsqldb/data directory.
- From the commmand line move to the hsqldb/data directory and run the following command to start the db:
java -cp ../lib/hsqldb.jar org.hsqldb.Server -database.0 file:mydb -dbname.0 learntechnology_db
- Copy the project-dir/external-resources/learntechnology-ds.xml file to JBOSS_HOME/server/default/deploy
- From the command line move to your project-dir and run "mvn clean install" (If you get some errors here make sure you installed maven and set you MAVEN_HOME and added MAVEN_HOME/bin to your environment path.)
- The above mvn command will have created several target directories and files in the submodules beneath your project-dir. Copy the project-dir/lt-ear/target/learntechnology-maven-jee=1.0.ear to JBOSS_HOME/server/default/deploy
- From the command line move to JBOSS_HOME/bin and run either the run.sh or run.bat file depending on your OS.
- Point your browser to http://localhost:8080/lt-web/ and click on the 'view users' link. You should see few users displayed in the resulting jsp.
NOTE ABOUT TESTING: mvn install will run the simple unit test. It also uses a log4j.properties file in test/resources and will output a log file ejb-jar-testing.log inside the lt-ejb module root. The test results will show up in lt-ear/target/surefire-reports. If you want to generate a nicer report, type mvn surefire-report:report from the command line. This will generate an html report called surefire-report.html under lt-ejb/target/site directory. (Running mvn site should generate that file as well, including a larger more complete report. See mvn docs for more info.)
The Project Structure
Here is how the project structure is laid out:
Basic:
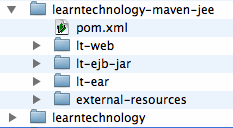
Expanded:
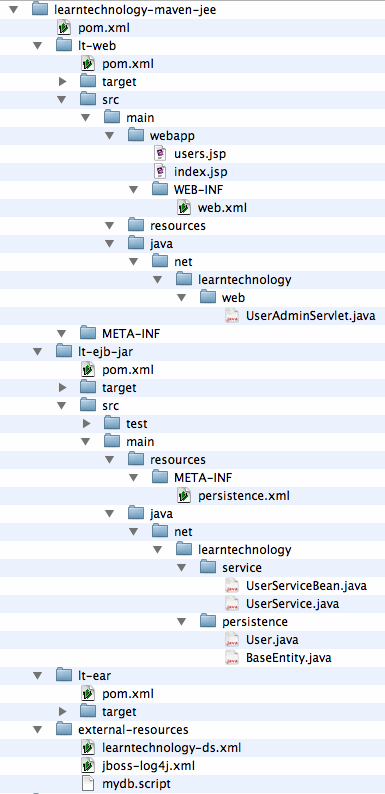
Basic:
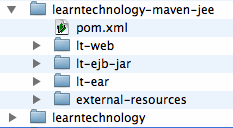
Expanded:
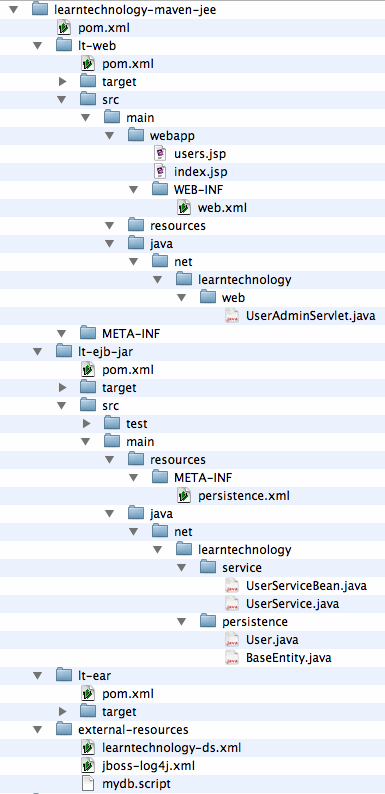
The pom.xml files
project parent pom.xml
4.0.0
net.learntechnology
learntechnology-maven-jee
1.0
pom
Sample Maven2 JEE Project
lt-ejb-jar
lt-web
lt-ear
maven2-repository.dev.java.net
Java.net Repository for Maven
http://download.java.net/maven/2/
default
org.apache.maven.plugins
maven-compiler-plugin
1.5
1.5
false
test.log
debug
true
log4j
log4j
1.2.13
provided
commons-collections
commons-collections
3.2
true
commons-lang
commons-lang
2.3
true
commons-logging
commons-logging
1.1.1
true
javax.ejb
ejb-api
3.0
provided
javax.persistence
persistence-api
1.0
provided
org.testng
testng
5.8
test
jdk15
ear pom.xml
4.0.0
net.learntechnology
learntechnology-maven-jee
1.0
net.learntechnology
lt-ear
1.0
ear
learntechnology-maven-jee :: lt-ear
learntechnology-maven-jee-${pom.version}
maven-ear-plugin
true
commons-collections
commons-collections
true
commons-logging
commons-logging
true
commons-lang
commons-lang
true
net.learntechnology
lt-ejb-jar
net.learntechnology
lt-web
/lt-web
codehaus snapshot repository
http://snapshots.repository.codehaus.org/
true
commons-collections
commons-collections
3.2
provided
commons-lang
commons-lang
2.3
provided
commons-logging
commons-logging
1.1.1
provided
net.learntechnology
lt-ejb-jar
ejb
1.0-SNAPSHOT
net.learntechnology
lt-web
war
1.0-SNAPSHOT
lt-ejb-jar pom.xml
4.0.0
net.learntechnology
learntechnology-maven-jee
1.0
net.learntechnology
lt-ejb-jar
1.0-SNAPSHOT
ejb
net.learntechnology :: lt-ejb-jar
src/test/resources
true
org.apache.maven.plugins
maven-ejb-plugin
3.0
org.apache.maven.plugins
maven-surefire-plugin
2.4.2
src/test/config/testng.xml
org.apache.maven.plugins
maven-surefire-report-plugin
2.4.2
src/test/config/testng.xml
commons-collections
commons-collections
3.2
provided
commons-lang
commons-lang
2.3
provided
org.hibernate
hibernate-entitymanager
3.2.0.ga
test
hsqldb
hsqldb
1.8.0.7
test
lt-web pom.xml
4.0.0
net.learntechnology
learntechnology-maven-jee
1.0
net.learntechnology
lt-web
1.0-SNAPSHOT
war
net.learntechnology :: lt-web war
src/main/resources
src/main/java
**/*.xml
src/test/resources
src/main/webapp
**/*.xml
javax.servlet
servlet-api
2.4
provided
javax.servlet
jstl
1.1.2
taglibs
standard
1.1.2
javax.ejb
ejb-api
3.0
provided
javax.persistence
persistence-api
1.0
provided
net.learntechnology
lt-ejb-jar
ejb
1.0-SNAPSHOT
provided
Relevant Files
Here are the more pertinent files used in the main application:
persistence.xml
org.hibernate.ejb.HibernatePersistence
java:/db/learntechnology
net.learntechnology.persistence.User
Base Entity
|
User Entity
|
UserService
@Local
|
UserServiceBean
@Stateless
|
UserAdminServlet
public class UserAdminServlet extends HttpServlet {
|
Relevant Testing Files
testng.xml
persistence.xml
<!-- <property name="hibernate.hbm2ddl.auto" value="create-drop"/> -->
org.hibernate.ejb.HibernatePersistence
${project.build.directory}/classes
BaseTest.java
public class BaseTest {
|
UsersTest.java
@Test(groups={"unit"})
|